Earlier this month on Friday, August 2, I attended my first developer conference. I had been looking for more learning opportunities and when I heard that there was going to be a conference somewhat local to me, I thought it would be a good experience for me to take part in. I could learn from multiple speakers on a variety of topics within a day. With only an hour for each talk, I knew I wasn't going to walk away with fully knowing a new skill or two but possibly I could take away enough to motivate me to seek out more information about some of them.
So I decided to attend the first JavaScript and Friends conference in Columbus, Ohio. JS&Friends is a not-for-profit conference that uses its proceeds to help cultivate the local (and remote) developer community in education and inclusivity. The schedule was packed with a lot of interesting talks about everything JavaScript - it was hard to choose which sessions to attend.
Starting the morning
Early Friday morning came and I was on the road to the Quest Conference Center off the far side of the loop (I-270 around Columbus). Arriving a bit early, the organizers let me know to help myself to breakfast while they get the attendee badges sorted. Great job on that, by the way! 👍️ Props to the fruit selection. I got my badge shortly after and waited for the welcome message to everyone before going to my first session. I made some notes on the session sheet they gave me for a possible plan of where to go. I still wasn't 100% sure which ones I was going to but was narrowing it down.
Below are summaries of the sessions I did attend. To trim down overall length, the more detailed and technical information can be found in expandable blocks in some sections.
Jump to:
- Pros & Cons of Vue vs React - Milu Franz
- Mastering Shadow DOM - Martine Dowden
- Test Them JavaScripts - Rob Tarr
- Lunch Keynote - Guy Royse
- Querying NoSQL with SQL - Matthew Groves
- Creating HTML tags with Vue & Web Components - Joe Erickson
- DevOps+IoT+Security - Diana Rodriguez
Milu Franz
Pros & Cons of Vue vs React -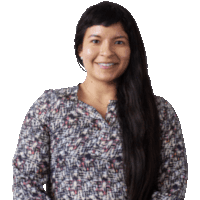
Milu Franz of Mile Two gave a great presentation comparing the advantages and drawbacks of both Vue and React. I'm novicely versed in building with Vue but I was interested in how it compares to the more popular React library.
Detailed notes
She showed that Vue is a progressive framework which includes official packages for state management and routing, whereas React is a library concentrating on UI development but has external third party packages for state management and routing. Also she explained the differences between Vue's template syntax, where it combines the markup, logic, and styling (as separate sections) in one single file component, and React's format, where markup is included inside the JavaScript code as JSX and styling is imported from an external .css file (unless using CSS-in-JS). Note that Vue components can also be modeled in the same fashion as React ones, if desired for more intricate customization, but the template syntax is a preferable method of designing most types of components.
Milu went over building the same To Do List project in both Vue & React, going over Vue's concepts of using interpolation in the markup (to insert JavaScript) and directives such as v-model for two-way data binding of state (that really can be described as magical). This is compared to React where explicit defining of state (through the useState hook) is required. The key differences are that Vue abstracts handling state so you only need to directly update a value (versus using a function to update it) and that Vue will only re-render what changed while React might re-render everything in that component's subtree structure.
Lifecycle method differences were explained as well as React's useEffect hook. Run-time performance was also compared with both being more performant depending on the use case. Milu even went over drawbacks to each: Vue with potential over-flexibility and lack of resources; and React with its steep learning curve, lack of native libraries, and difficulty in collaborating with designers (harder to translate to markup when JSX is required).
My take from the presentation was that React is still more popular - it's been out longer and there's something that captivates developers to write it all in JavaScript, but Vue is catching up in terms of maturity, performance, and use cases. Both are fully able to help developers create engaging applications. I'm more of a Vue fan, obviously, but I can see why developers are attracted to it for their projects. For me, though, I really like the template markup as I can more easily visualize the layout without all the JavaScript around it. I also don't mind that Vue handles the state so magically for me. Abstract it away and make the process easier.
I'm sure there are plenty of other technical differences and opinions that make one better than the other but I look at it like I do with other technologies: Use what you like and what works. And keep the solution simple.
Martine Dowden
Mastering Shadow DOM -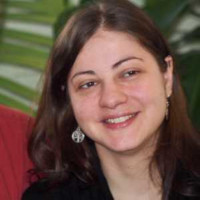
Martine showed us how to create web components using just HTML and vanilla JavaScript - that's right, no framework!
We learned what Web Components and Custom Elements are as well as how the Shadow DOM works to give us the ability for encapsulated reusable components. After reviewing basic DOM manipulation with JavaScript, she showed us how to create a web component using those techniques by bringing back the <blink>
tag!
Detailed notes
Defining our tag
JavaScript
// blink.js
const Blink = (function() {
"use strict";
class Component extends HTMLElement {
constructor() {
super();
// Create shadow root
const shadow = this.attachShadow({
mode: "open"
});
// Wrap text content with a span tag
const wrapper = document.createElement("span");
// Make a slot for text
const slot = document.createElement("slot");
slot.setAttribute("name", "content");
wrapper.appendChild(slot);
// CSS animation styles
const style = document.createElement("style");
style.textContent = `
@keyframes blink {
0% { visibility: hidden; }
50% { visibility: hidden; }
100% { visibility: visible; }
}
/* :host selects shadow root host */
:host { animation: 1s linear infinite blink; }
`;
// Append
shadow.appendChild(wrapper);
wrapper.appendChild(style);
}
}
customElements.define("wc-blink", Component); // can't use <blink> - it's still reserved
})();
export { Blink };
Putting it to use
HTML
<script>
import { Blink } from "./blink.js";
</script>
<!-- prettier-ignore -->
<wc-blink>
<h1 slot="content">
Look Ma, I'm blinking!
</h1>
</wc-blink>
I learned a bunch in this session on how the Shadow DOM is used to make custom elements and the benefits & limitations of such. I walked away with an understanding that it is possible to create a component-based site/application without a framework if need be. Though certainly the additional features of a framework may outweigh the freedom of independence, it is good to know there are options.
Rob Tarr
Test Them JavaScripts -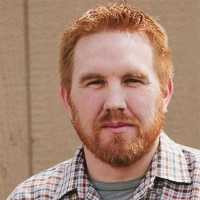
Rob of Sparkbox started his discussion on testing with a few questions for us:
- What does testing mean to your team?
- How do you test?
- How often do you test?
Some answers:
- Piece of mind, consistency
- Manually in browser, while developing (TDD), with testing libraries
- Rarely, all the time, during CI, before pushing to repository
He continued with why we test our code. It does give us piece of mind & consistency as well as giving confidence in the code and ourselves & designing better software because of it. But where do we start - especially when web development has had a culture not testing for so long? For whatever reasons, it's hard, there's no time or it's just an afterthought.
Rob listed out a few testing frameworks: Mocha, Ava, Jest, & Jasmine; though he was going to concentrate on Mocha (with the Chai add-on) for this short session. He mentioned different runners to use to initiate the tests: Grunt, Gulp, Karma, Make, npm.
Establishing boundaries of what to test is a very important point so that time is not wasted testing things that were (probably) already tested. He called it the concept of mowing your own yard. Don't test libraries and APIs. Just test your code.
I believe there was supposed to be more to the talk and possibly examples of how to start writing the tests - where do I begin? But with only 50 minutes available, it may have been cut short of that. I am aware of my knowledge gap in automated testing and I will definitely educate myself more on it.
Guy Royse
Lunch Keynote -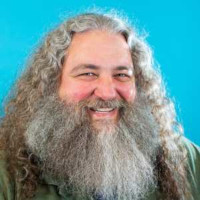
During lunch, Guy gave a short informal talk on the linguistics of code, specifically how programming is mainly in English and how code routines would look if they were in another language, such as Spanish.
Not only changing the words but the sentence structure as well. He pointed out that our function calls also follow the English structure, i.e. noun.verb(object). He then showed us in detail how we could translate our a common FizzBuzz function into Latin.
It was an amazing ride. I'd still like to see a framework coded in a Yoda-based structure (object(noun).verb ??).
Matthew Groves
Querying NoSQL with SQL -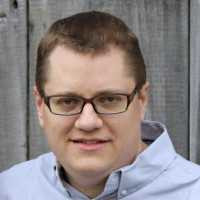
I had always wanted to read up more on NoSQL databases and how they differ from relational databases. I have worked with SQL for 14 years so the relational design & structure is strong in how I think of table schema.
Matthew explained the benefits of using NoSQL over SQL: scalability (spin up more servers or cluster them if needed), flexiblity (no strict schema needed), and performance. He noted that we don't have to totally abandon relational databases - they can coexist.
Detailed notes
While relational databases link separate tables by foreign keys, the JSON document model (the format NoSQL uses) can place related data in the same document as the main record. These extra pieces might be array objects if it contains more than one instance of child data. Other documents for seperate concepts can be created and referenced by a key.
Once he explained the concepts behind NoSQL, he showed us that queries (very similar to SQL's) can be created to find data. This is called N1QL (pronounced nickel) which is a Couchbase-centric query language. Azure Cosmos has something similar as well. Other NoSQL platforms would need to use aggregate functions which, to me, don't look very readable at first glance.
SELECT field
FROM `bucket` /* buckets contain logical groups of data-items */
WHERE otherfield = somevalue
/* JOINs are possible too! */
JOIN `otherbucket` ON (bucket.id = META(otherbucket).id)
LIMIT number;
N1QL queries return JSON objects that we can parse in our native coding language.
If there's anything to take away from this talk, Matthew says it's these 3 things:
- Pick the right application. Whether it's NoSQL or SQL, use what makes sense.
- JSON data is modeled differently. The structure of a document may be very different than a table.
- Query NoSQL data with SQL. So much easier to write.
Joe Erickson
Creating HTML tags with Vue & Web Components -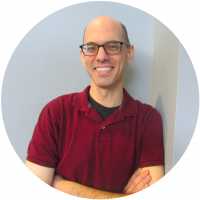
Joe of Tech Elevator gave a talk about creating web components. Similar to a previous talk; however, Joe's spin on it involved creating the them using Vue.
Joe flashed us back to 1997:
- Deep Blue beats Kasparov
- Microsoft buys a stake in Apple, saving the company
- Harry Potter and the Philosopher's Stone was published
- All major browsers supported the
<blink>
tag
And he intended that day to bring it back, at least for right then. Joe went through the steps to scaffold the initial code with Vue CLI and write out the component itself (using a different method of creating the blink animation).
Detailed notes
Creating the component
Using Vue's template syntax, the component can be written in separate blocks for markup, logic, and styling.
Blink.vue
<template>
<span ref="blinkyText"><slot /></span>
</template>
<script>
export default {
name: "blink",
props: {
interval: {
type: Number,
default: 500
}
},
mounted() {
setInterval(() => {
this.$refs.blinkyText.classList.toggle("onoff");
}, this.interval);
}
};
</script>
<style>
.onoff {
visibility: hidden;
}
</style>
Building the component
By default Vue CLI sets its scripts to build an application. The build script needs to be tweaked a little.
package.json
...
"scripts": {
"serve": "vue-cli-service serve",
"build": "vue-cli-service build --target wc --name my-blink 'src/components/Blink.vue'",
"lint": "vue-cli-service lint"
},
...
Now npm run build
can be executed and it will be created in the dist folder. Inserted the component into a page entails including the blink.js file as well as the Vue script - and don't forget the tag itself!
<meta charset="utf-8" />
<title>my-blink demo</title>
<script src="https://unpkg.com/vue"></script>
<script src="./my-blink.js"></script>
<my-blink interval="1000">Blink every second</my-blink>
Joe also gave us examples of real and useful components that he has made, how they can interact with each other, and how to build them all at once. And just because they were built with Vue doesn't mean they have to be used in a completely Vue-made app. They can be used in a plain HTML page as long as the Vue script is called.
While the discussion and concepts were similar to Martine's, knowing there is a different way to create a component that can be used freely in non-frameworked sites is great to know.
Diana Rodriguez
DevOps+IoT+Security -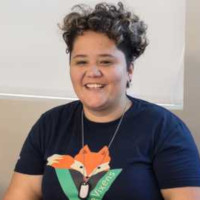
Diana delivered a very insightful talk about the link between DevOps, IoT (Internet of Things), and security. It comes down to collaboration and feedback.
DevOps is about collaboration between development and operations. Diana gave us this passage from the Vue.js docs regarding the effects of DevOps:
The adoption of DevOps culture, tools and agile engineering practices has, among other things, the nice effect of increasing the collaboration between the roles of development and operations. One of the main problems of the past (but also today in some realities) is that the dev team tended to be uninterested in the operation and maintenance of a system once it was handed over to the ops team, while the latter tended to be not really aware of the system’s business goals and, therefore, reluctant in satisfying the operational needs of the system (also referred to as “whims of developers”).
What this means is that DevOps works to bring solutions together for the benefit of the development and operations teams. For example, they may suggest using containers (like Docker) to limit the differences between the environment of a developer's laptop and the production server.
She explained that efficient DevOps will also provide faster feedback which bring about quicker & better enhancement/repairs as well as insight around performance and usage. With IoT, feedback regarding device performance and usage is analyzed and used to tune the product's quality for everyone's benefit.
The part of Diana's talk regarding security was eye-opening.
There is a huge lack of faith in the security of IoT devices by not only customers but developers too.
Of those surveyed:
- 52% believe that most IoT devices on the market right now DO NOT have the necessary security in place
- 49% don’t trust having their personal/private data tied to IoT devices – but still use them
- Only 18% trust having their personal data tied to IoT devices
- Nearly 35% claimed that the breaches of major companies have not had much of an effect on the trust or consumer interest in these brands
- 85% of developers surveyed have felt rushed to get an application to market due to demand/pressure in the last 6 months
- 90% of developers surveyed do not believe that IoT devices on the market currently have the necessary security in place
Not only IoT devices for smart homes are potentially security-light but wearables as well. Peoples' biometric data is out there in the cloud which can sound kind of scary. Imagine how you would feel in the case of a data breach. 😱 How much personal information and security are people willing to unknowingly give out in the name of convenience?
Why aren't more developers/companies focused more on security instead of delivering a product quickly? The answer is mostly about money unfortunately. Companies also deter from seeking outside consultation because people don't like being told what they're doing wrong.
Diana then gave suggestions on what to concentrate on for increased device security: secure update processes, authentication, encryption, & independent security assessments to name a few.
At the end there were a few questions to ask yourself regarding your own IoT usage.
- What devices are we using?
- What information do we share?
- What information do we have access to?
It was a very interesting presentation focusing on collaboration, capturing valuable feedback, and being security-minded. There was so much information - one of the best talks that day.
Mentoring Panel & Closing
After the last session, JS&Friends hosted a panel of speakers who gave their insights and experiences on mentoring, answering questions from the rest of us. There were great questions and answers, unfortunately I cannot remember them in detail - I just know it was a good and helpful discussion. I was so focused on listening that I didn't take any notes.
In closing, my first developer conference was a very interesting experience and I am glad I was able to attend! I was able to hear a variety of topics discussed, networked a bit, learned a few things, and most importantly - I got out of my comfort zone of introversion, at least for a little bit. I hope to be at next year's JS&Friends Conference and any others that come along near me.